Quick Start
This short tutorial is going to show you how to get started scripting in Multiverse using Lua. To avoid any confusion, I would recommend using a clean Multiverse install, and to keep things even simpler, we're going to be focusing on adding Lua scripting support to the original The Beginning campaign.
Contact
Having trouble getting started? Contact me at toxicity@thebeginning.uk
Requirements​
- Working installation of Populous: The Beginning.
- Working installation of the Multiverse Launcher v3.3.8 or higher.
- Campaign pack Populous: The Beginning installed.
- Any text editor, but I highly suggest using Notepad++.
The Process​
Open the Multiverse Launcher and move your cursor to the right side – where the campaign list is shown. Hover over the "Populous: The Beginning" entry and right click it, then select "Open Directory". A new Windows File Explorer should have opened. This is the installation folder of the campaign pack.
From this directory location, we want to open "info.json" inside a text editor, where we can now change the Lua
field to true
.
NOTE: After we save our changes, we absolutely want to restart the Multiverse Launcher.
"Version": "Final",
"ReleaseDate": "May 17, 1998",
"Levels": 25,
"Lua": true,
"SoundEngine": false,
"Future1": -1,
Moving forward, in the root campaign directory we have to make a new folder titled "Scripts" – the location where, shockingly, our scripts will reside.
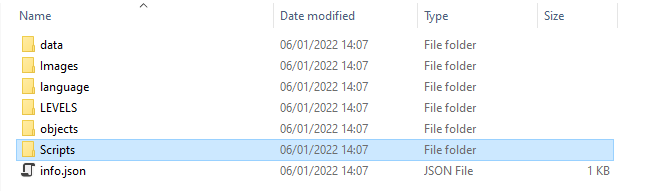
Open the newly created folder, then create a new blank text file inside. Open the text file, go to File
and select Save As
and give it the name "main.lua". Repeat the saving process once again, but this time name it "level_1.lua".
Let's open main.lua inside a text editor. This, as the name suggests, is the core Lua file. It will be automatically loaded by the game on start-up, and it is considered the Parent
script which remains active until the game is closed. We'll use it to load additional scripts, using the AddScript
function, which loads Child
scripts. Unlike the Parent
script, these will be de-activated once the user exists or changes a level.
NOTE: It's important to double check the extension type. It has to be ".lua"!
Now, as mentioned above, let's write a piece of code that will load the scripts for us when neccessary. We're going to be using the OnScript event to do so.
function OnScript(level)
-- Check whether the user has selected level 1.
if (level == 1) then
-- Now load the additional script!
AddScript("level_1.lua")
end
end
Done! After saving our changes, we're going to be switching focus to level_1.lua, the other file we just made.
Let's write something simple and quick, like a lava trail that follows our Shaman.
function OnTurn()
-- Create a local variable and store a reference to our Shaman into it.
-- The function getShaman() will return a nil value if our shaman does not exist.
-- It's important to check for invalid Thing* objects when we interact with them,
-- otherwise the game will crash. We will make use of the variable "shaman" below.
local shaman = getShaman(TRIBE_BLUE)
-- Ensure it's a valid object.
if (shaman ~= nil) then
-- Create a lava square effect at our Shaman position.
create_thing(T_EFFECT, M_EFFECT_LAVA_SQUARE, TRIBE_BLUE, shaman.Pos.D3)
-- Create a fireball effect at our Shaman position.
create_thing(T_EFFECT, M_EFFECT_FIREBALL, TRIBE_BLUE, shaman.Pos.D3)
end
end
Result​
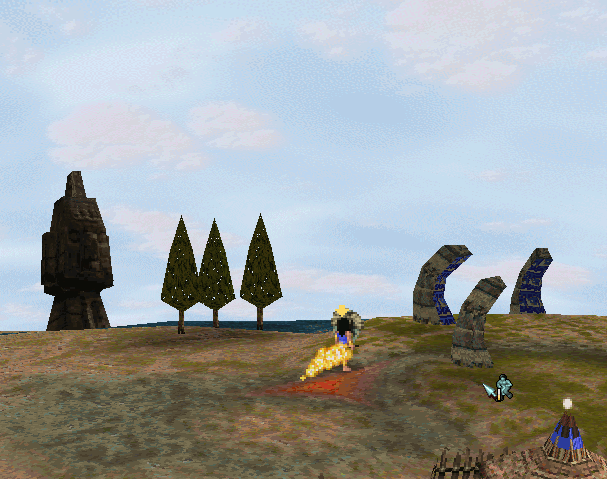